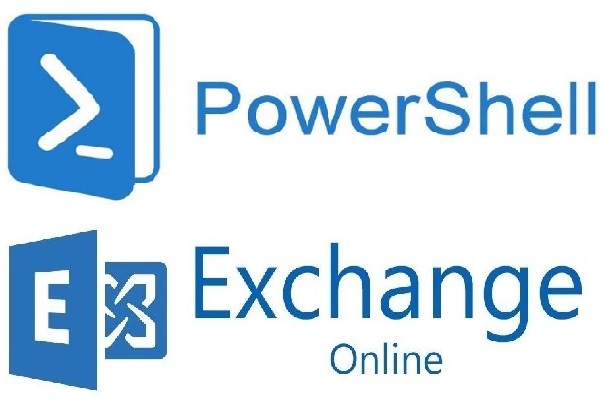
Transport Rule Properties updates using PowerShell
In this blog I will share one way to write a function to update, add or remove values, a specific property of an Exchange Online transport (mail flow) rule using PowerShell.
Note: Function included in this post works with parameters accept string[].
Introduction
My blogs have relatively simple, and sometimes complex, examples. I’m hoping that you will be able to tailor them to your need or use them in your own scripts.
The goal of this blog is to show one way to accomplish a task. It is not to show how to write a perfect script, solution or process to accomplish a task.
Prerequisites
- First thing to remember is to install Azure PowerShell. Otherwise, you can use Cloud Shell if you prefer to stay within Azure Portal.
- Secondly, install Install the EXO V2 module. Otherwise, you can use Cloud Shell if you prefer to stay within Azure Portal.
PowerShell Cmdlets
- Connect-ExchangeOnline Used in the Exchange Online PowerShell V2 module to connect to Exchange Online PowerShell using modern authentication. This cmdlet works for MFA or non-MFA enabled accounts.
- Set-TransportRule Use to view transport rules (mail flow rules) in your organization.
Connect to Exchange Online
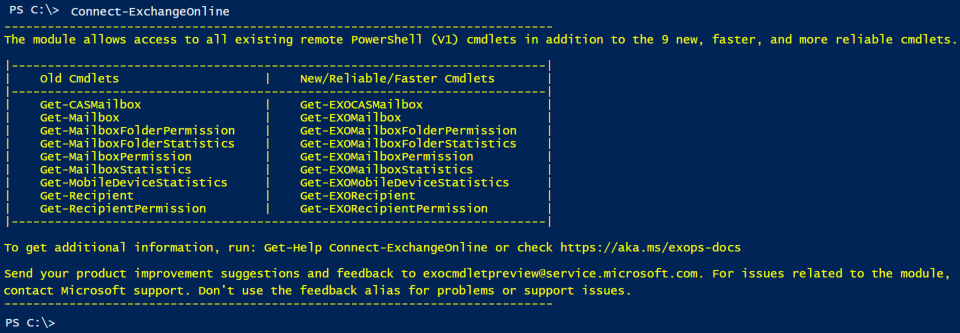
Get a transport (mail flow) rule properties
In its simplest form.
1 2 3 4 |
$RuleName = "External Emails Notice" Set-TransportRule -Identity $RuleName -ExceptIfSenderDomainIs apple.com,microsoft.com # Or Set-TransportRule -Identity $RuleName -ExceptIfSenderDomainIs "apple.com","microsoft.com" |
For the cmdlet parameters values, you can enter multiple values separated by commas. Use quotation marks if the values contain spaces: “Value1″,”Value2″,…”ValueN”. Review Set-TransportRule.
Why a function is needed
- Connect to Exchange Online if a connection is not yet established.
- Stop and display a single line error message if the transport rule does not exist.
- Accept pipeline input.
- Add the code to a library to be loaded at PowerShell startup.
- other reasons that I already forgot.
Let us have fun and start writing our function.
Our Set-TransportRulePropertiesS function
Firstly, set up function parameters including pipeline input
The function will have four parameters: (1) transport rule name and (2) property to be set/updated (3) values to be added (4) values to be removed.
1 2 3 4 5 6 7 8 9 10 11 |
[CmdletBinding()] Param ( [Parameter(Mandatory=$true, HelpMessage="Enter rule name")] [String] $RuleName, [Parameter(Mandatory=$true, HelpMessage="What part of the rule to update, e.g. From, ExceptIfSenderDomainIs, etc.")] [String] $Property, [Parameter(Mandatory=$false, HelpMessage="What to add to the rule")] [String[]] $AddValues = "", [Parameter(Mandatory=$false, HelpMessage="What to remove from the rule")] [String[]] $RemoveValues = "" ) |
Secondly, Connect to Exchange Online if a connection is not established
1 2 3 |
$GetSessions = Get-PSSession | Select-Object -Property State, Name $IsConnected = (@($GetSessions) -like '@{State=Opened; Name=ExchangeOnlineInternalSession*').Count -gt 0 If ($IsConnected -ne "True") { Connect-ExchangeOnline } |
Thirdly, Check if transport rule exist
Save existing property values in $NewValues variable.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
# Next two lines are used to test this code snippet $RuleName = "External Emails Notice" $Property = "ExceptIfSenderDomainIs" $Rule = Get-TransportRule $RuleName -ErrorAction SilentlyContinue If ($Rule -eq $null) { Write-Host -ForegroundColor Red "`nRule [$RuleName] does not exist" Break } Else { [System.Collections.ArrayList] $NewValues = $Rule.$Property } # Next line is used to test this code snippet $NewValues | Sort-Object |
Fourthly, Code snippet to add values to a property
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
# Next line is used to test this code snippet [String[]] $AddValues = "microsoftonline.com","office365.com" Write-Host "" ForEach ($AddValue in $AddValues) { Write-Host "Adding [$AddValue]" $NewValues = ($NewValues + $AddValue) | Select -Unique } # The following lines are used to test this code snippet Write-Host "Updating [$RuleName][$Property]" -ForegroundColor DarkYellow $Parameters = @{$Property = $NewValues} Set-TransportRule -Identity $RuleName @Parameters $Rule = Get-TransportRule $RuleName -ErrorAction SilentlyContinue [System.Collections.ArrayList] $NewValues = $Rule.$Property $NewValues | Sort-Object |
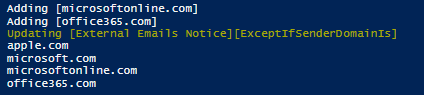
Finally, Code snippet to remove values from a property
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
# Next line is used to test this code snippet [String[]] $RemoveValues = "apple.com","office365.com","ccsend.com1" Write-Host "" ForEach ($RemoveValue in $RemoveValues) { If ($NewValues -contains $RemoveValue) { Write-Host "Removing [$RemoveValue]" $NewValues.Remove($RemoveValue) } Else { Write-Host "Value [$RemoveValue] does not exist in property [$Property]" -ForegroundColor Red } } # The following lines are used to test this code snippet Write-Host "Updating [$RuleName][$Property]" -ForegroundColor DarkYellow $Parameters = @{$Property = $NewValues} Set-TransportRule -Identity $RuleName @Parameters $Rule = Get-TransportRule $RuleName -ErrorAction SilentlyContinue [System.Collections.ArrayList] $NewValues = $Rule.$Property $NewValues | Sort-Object |

Without delay, our complete amazing Set-TransportRulePropertiesS function
Putting all the pieces together produce a nice function. What’s more, you can add Comment-based Help for a Function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
Function Set-TransportRulePropertiesS { [CmdletBinding()] Param ( [Parameter(Mandatory=$true, HelpMessage="Enter rule name")] [String] $RuleName, [Parameter(Mandatory=$true, HelpMessage="What part of the rule to update, e.g. From, ExceptIfSenderDomainIs, etc.")] [String] $Property, [Parameter(Mandatory=$false, HelpMessage="What to add to the rule")] [String[]] $AddValues = "", [Parameter(Mandatory=$false, HelpMessage="What to remove from the rule")] [String[]] $RemoveValues = "" ) Begin { #Connect & Login to ExchangeOnline (MFA) $GetSessions = Get-PSSession | Select-Object -Property State, Name $IsConnected = (@($GetSessions) -like '@{State=Opened; Name=ExchangeOnlineInternalSession*').Count -gt 0 If ($IsConnected -ne "True") { Connect-ExchangeOnline } $Rule = Get-TransportRule $RuleName -ErrorAction SilentlyContinue If ($Rule -eq $null) { Write-Host -ForegroundColor Red "`nRule [$RuleName] does not exist" Break } Else { [System.Collections.ArrayList] $NewValues = $Rule.$Property } } # Begin Process { <# $RuleName = "Phishing emails, Move to Spam folder" $Property = "SubjectOrBodyMatchesPatterns" # "From" $emailToBlock = "Sее аttаchеd rеmittаncе" # "cindy.roberts@metamaterial.com" #> Write-Host "" If ($AddValues -eq "" -and $RemoveValues -eq "") { Write-Host -ForegroundColor Cyan "No value to add or remove. So, nothing to do." } Else { If ($AddValues -ne "") { ForEach ($AddValue in $AddValues) { Write-Host "Adding [$AddValue]" $NewValues = ($NewValues + $AddValue) | Select -Unique } } If ($RemoveValues -ne "") { ForEach ($RemoveValue in $RemoveValues) { If ($NewValues -contains $RemoveValue) { Write-Host "Removing [$RemoveValue]" $NewValues.Remove($RemoveValue) } Else { Write-Host "Value [$RemoveValue] does not exist in property [$Property]" -ForegroundColor Red } } } Write-Host "Updating [$RuleName][$Property]" -ForegroundColor DarkYellow $Parameters = @{$Property = $NewValues} Set-TransportRule -Identity $RuleName @Parameters $Rule = Get-TransportRule $RuleName } } # Process End { Return ($Rule.$Property | Sort-Object) } # End } # Set-TransportRulePropertiesS #endregion |
Putting our amazing function to work
Examples to illustrate the usage of our amazing Get-TransportRulePropertiesS function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
# Add values Set-TransportRulePropertiesS -RuleName "External Emails Notice" ` -Property "ExceptIfSenderDomainIs" ` -AddValues "microsoftonline.com","office365.com" # Remove values Set-TransportRulePropertiesS -RuleName "External Emails Notice" ` -Property "ExceptIfSenderDomainIs" ` -RemoveValues "apple.com","office365.com","ccsend.com1" # Add and remove values Set-TransportRulePropertiesS -RuleName "External Emails Notice" ` -Property "ExceptIfSenderDomainIs" ` -AddValues "microsoftonline.com","office365.com" ` -RemoveValues "apple.com","office365.com","ccsend.com1" |
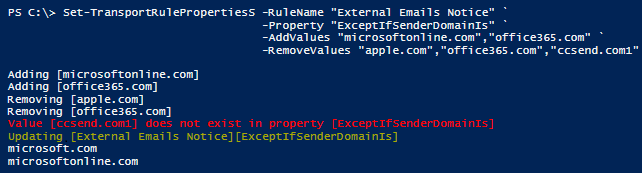
In conclusion
In summary, we explored writing a function to update Transport Rule properties that accepts String[] using PowerShell. We also connected to Exchange Online if there was no connection. And also if the rule does not exist, an error message is displayed.
Note: Function works with parameters accept string[].
Did you find this blog easy to follow and helpful to you? I certainly would love to hear your feedback and suggestions. So, let me know in the comments below. Happy PowerShelling.
Disclaimer
Purpose of the code contained in blog is solely for learning and demo purposes. Author will not be held responsible for any failure or damages caused due to any other usage.
Right on! It was very difficult to find that each rule has conditions that need to be queried by name. Lots of searching before i found your script. Even ChatGPT is not aware of these options yet.
Thanks!!!
Great, I’m glad this script was helpful to you.
Is there a way/script to go through each transport rule looking for a UPN and remove UPN from it?
Yes, the function can be modified to accommodate this option.
A quick solution would be to query all existing transport rules then use a ForEach with the above function to remove UPN.
Here is an example:
$RemoveUPN = “removeme.com”
$TransportRules = Get-TransportRule -ResultSize Unlimited
ForEach ($TransportRule in $TransportRules) {
# Remove values
Set-TransportRulePropertiesS -RuleName $TransportRule.Name
-Property "ExceptIfSenderDomainIs"
-RemoveValues $RemoveUPN
}
I hope I answered your question. Good luck.