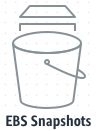
Get all AWS EBS snapshots across all profiles and regions
In this blog, I will share a script to retrieve all AWS EBS snapshots across all profiles and regions, along with associated volume and EC2 information where available. You can choose to display the results, export them to a file, or both.
For each snapshot, I will retrieve the following attributes: Profile, Region, SnapshotId, Name, Encrypted, VolumeId, VolumeName, VolumeStatus, EC2InstanceId, EC2Name, and EC2State. You can also retrieve other attributes if needed.
Explore my other articles about AWS services:
- AWS IAM role is not listed in the IAM roles dropdown menu for EC2
- Get all AWS Backup protected resources across all profiles and regions
- Get all AWS Backup recovery points grouped by resource name across all profiles and regions
- Get all AWS EC2 instances across all profiles and regions
Introduction
Amazon EBS snapshots You can back up the data on your Amazon EBS volumes by making point-in-time copies, known as Amazon EBS snapshots.
I required a summary list of EBS snapshots across all profiles and regions, along with associated volume and EC2 information where available. To accomplish this, I utilized PowerShell and AWS Tools for PowerShell.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<# Requested EBS snapshot information, along with associated volume and EC2 information. Profile : Production Region : us-east-1 SnapshotId : snap-0a4699ca123456789 Name : PRD-FILESRV01 - Accounting Encrypted : True VolumeId : vol-0a4699ca123456789 VolumeName : PRD-FILESRV01-Accounting-/dev/sda1 VolumeStatus : in-use EC2InstanceId : i-0a4699ca123456789 EC2Name : PRD-FILESRV01 EC2State : Running #> |

Prerequisites
To execute the script provided in this blog, you need to:
- Install and configure AWS Tools for PowerShell.
- Log in to all necessary AWS accounts and profiles.
- Configure your IAM permissions to allow access to Amazon EC2.
PowerShell Cmdlets
Here are the PowerShell cmdlets we will use.
- Get-AWSCredential Returns an AWSCredentials object initialized with from either credentials currently set as default in the shell or saved and associated with the supplied name from the local credential store.
- Get-Culture Gets the current culture set in the operating system.
- Get-EC2Instance Describes the specified instances or all instances.
- Get-EC2Region Describes the Regions that are enabled for your account, or all Regions.
- Get-EC2Snapshot Describes the specified EBS snapshots available to you or all of the EBS snapshots available to you.
- Get-EC2Volume Describes the specified EBS volumes or all of your EBS volumes.
Retrieve EBS snapshots within a single profile and region
First, I used Get-EC2Snapshot cmdlet to retrieve EBS snapshots within a single profile and region.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
$Profile = "Production" $Region = "us-east-1" $Snapshots = Get-EC2Snapshot -ProfileName $Profile ` -Region $Region ` -OwnerId self $Snapshot = $Snapshots | Select -First 1 $Snapshot <# $Snapshot DataEncryptionKeyId : Description : Created by CreateImage(i-09df6dca6ea9ebaca) for ami-0cbcdfab99f5d3c64 Encrypted : True KmsKeyId : arn:aws:kms:us-east-1:217123456789:key/12345678-51e4-48fb-b50a-9b3123456789 OutpostArn : OwnerAlias : OwnerId : 217123456789 Progress : 100% RestoreExpiryTime : 1/1/0001 12:00:00 AM SnapshotId : snap-0d04d22c123456789 SseType : StartTime : 3/9/2021 11:32:57 PM State : completed StateMessage : StorageTier : standard Tags : {Name} VolumeId : vol-0a4699ca123456789 VolumeSize : 100 #> |
Retrieve information about an EBS snapshot volume
Second, I used Get-EC2Volume cmdlet to retrieve information about an EBS snapshot volume.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
$Profile = "Production" $Region = "us-east-1" $Snapshots = Get-EC2Snapshot -ProfileName $Profile ` -Region $Region ` -OwnerId self $Snapshot = $Snapshots | Select -First 1 $Volume = Get-EC2Volume -ProfileName $Profile ` -Region $Region ` -VolumeId $Snapshot.VolumeId $Volume <# $Volume Attachments : {i-0a4699ca123456789} AvailabilityZone : us-east-1b CreateTime : 8/11/2023 9:37:29 PM Encrypted : True FastRestored : False Iops : 6000 KmsKeyId : arn:aws:kms:us-east-1:217123456789:key/12345678-51e4-48fb-b50a-9b3123456789 MultiAttachEnabled : False OutpostArn : Size : 100 SnapshotId : snap-0a4699ca123456789 SseType : State : in-use Tags : {Name} Throughput : 1000 VolumeId : vol-0a4699ca123456789 VolumeType : gp3 #> |
Retrieve information about an EBS snapshot volume attachments
I used the Get-EC2Instance cmdlet to retrieve information about EBS snapshot volume attachments. In my environment, each volume is connected to only one EC2 instance. I will retrieve only the EC2 name and its state.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
$Profile = "Production" $Region = "us-east-1" $Snapshots = Get-EC2Snapshot -ProfileName $Profile ` -Region $Region ` -OwnerId self` $Snapshot = $Snapshots | Select -First 1 $Volume = Get-EC2Volume -ProfileName $Profile ` -Region $Region ` -VolumeId $Snapshot.VolumeId $EC2 = (Get-EC2Instance -ProfileName $Profile ` -Region $Region ` -InstanceId $Volume.Attachments[0].InstanceId).Instances $EC2 | Select InstanceId, @{N="Name";E={$_.Tag.Value[$_.Tag.Key.IndexOf("Name")]}}, @{N="State";E={(Get-Culture).TextInfo.ToTitleCase($_.State.Name)}} <# $EC2 InstanceId Name State ---------- ---- ----- i-0a4699ca123456789 PRD-FILESRV01 Running #> |
Compile requested EBS snapshot information along with associated volume and EC2 details
Third, I used Get-EC2Instance cmdlet to retrieve information about an EBS snapshot volume.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
$Info = $Snapshot | Select @{N="Profile";E={$Profile}}, @{N="Region";E={$Region}}, SnapshotId, @{N="Name";E={($_.Tag | Where -Property Key -eq Name).Value}}, Encrypted, VolumeId, @{N="VolumeName";E={($Volume.Tag | Where -Property Key -eq Name).Value}}, @{N="VolumeStatus";E={$Volume.Status.Value}}, @{N="EC2InstanceId";E={$EC2.InstanceId}}, @{N="EC2Name";E={$EC2.Tag.Value[$EC2.Tag.Key.IndexOf("Name")]}}, @{N="EC2State";E={(Get-Culture).TextInfo.ToTitleCase($EC2.State.Name)}} $Info <# $Info - Compiled requested EBS snapshot information Profile : Production Region : us-east-1 Name : PRD-FILESRV01 - Accounting SnapshotId : snap-0a4699ca123456789 Encrypted : True VolumeId : vol-0a4699ca123456789 VolumeName : PRD-FILESRV01-Accounting-/dev/sda1 VolumeStatus : in-use EC2InstanceId : i-0a4699ca123456789 EC2Name : PRD-FILESRV01 EC2State : Running #> |
Compile requested EBS snapshot information within a single profile and region
Fourth, I will iterate through the above steps for each EBS snapshot.
- To expedite processing, I will fetch all available EC2 instances and volumes within the specified profile and region, then filter them based on the snapshot criteria.
- Additionally, I will address cases where the EBS snapshot volume does not exist and where the EBS snapshot volume attachment does exist.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 |
$CsvFile = "C:\Temp\Snapshotinfo.csv" $Info = @() # Compiled requested EBS snapshot information $Profile = "Production" $Region = "us-east-1" Write-Host("Profile: $Profile") Write-Host("`tRegion: $Region") # Get snashots. Write-Host ("`t`tRetrieving snapshots...") $Snapshots = Get-EC2Snapshot -ProfileName $Profile ` -Region $Region ` -OwnerId self If ($Snapshots) { # Get volumes. Write-Host ("`t`tRetrieving volumes...") $Volumes = Get-EC2Volume -ProfileName $Profile -Region $Region # Get EC2s Write-Host ("`t`tRetrieving EC2s...") $EC2s = (Get-EC2Instance -ProfileName $Profile -Region $Region).Instances Write-Host("`t`tsnapshots count: $($Snapshots.Count)") # For each snapshot: $Count = 1 ForEach ($Snapshot in $Snapshots) { Write-Host ("`t`t`t$Count- $($Snapshot.SnapshotId)") # Get Volume information. If ($Count -eq 4) { break } $Volume = $Volumes | Where {$_.VolumeId -eq $Snapshot.VolumeId} If ($Volume) { $VolumeExists = "Yes" $VolumeName = ($Volume.Tag | Where -Property Key -eq Name).Value $VolumeStatus = $Volume.Status.Value # Get attached EC2 information. If ($Volume.Attachment) { $EC2 = $EC2s | Where {$_.InstanceId -eq $Volume.Attachments.InstanceId} $EC2InstanceId = $EC2.InstanceId $EC2Name = ($EC2.Tag | Where -Property Key -eq Name).Value $EC2State = (Get-Culture).TextInfo.ToTitleCase($EC2.State.Name) } } Else { $EC2InstanceId = "" $EC2Name = "" $EC2State = "" $VolumeExists = "No" $VolumeName = "" $VolumeStatus = "" } $Info += $Snapshot | Select @{N="Profile";E={$Profile}}, @{N="Region";E={$Region}}, SnapshotId, @{N="Name";E={($_.Tag | Where -Property Key -eq Name).Value}}, Encrypted, VolumeId, @{N="VolumeName";E={$VolumeName}}, @{N="VolumeStatus";E={$VolumeStatus}}, @{N="EC2InstanceId";E={$VolumeStatus}}, @{N="EC2Name";E={$EC2Name}}, @{N="EC2State";E={$EC2State}} $Count++ } # $Snapshot } Else { Write-Host("`t`tsnapshots count: 0") } $Info | Export-Csv -NoTypeInformation -Path $CsvFile <# Profile: Production Region: us-east-1 Retrieving snapshots... Retrieving volumes... Retrieving EC2s... snapshots count: 4 1- snap-0d04d22c123456789 2- snap-0d04d22c123456781 3- snap-0d04d22c123456782 4- snap-0d04d22c123456783 #> |
Compile requested EBS snapshot information across all profiles and enabled regions
Finally, I will iterate through the aforementioned steps across all profiles and enabled regions.
- To expedite processing, I will fetch all available EC2 instances and volumes within the specified profile and region, then filter them based on the snapshot criteria.
- Additionally, I will address cases where the EBS snapshot volume does not exist and where the EBS snapshot volume attachment does exist.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 |
$CsvFile = "C:\Temp\Snapshotinfo.csv" $DefaultRegion = "us-east-1" $Info = @() # Compiled requested EBS snapshot information # Get profiles. $Profiles = (Get-AWSCredential -ListProfileDetail | ` Where-Object {$_.ProfileName -notlike "default"} | ` Sort-Object ProfileName).ProfileName ForEach ($Profile in $Profiles) { Write-Host("Profile: $Profile") # Get enabled regions. $EnabledRegions = ` (Get-ACCTRegionList -ProfileName $Profile ` -Region $DefaultRegion ` -RegionOptStatusContain @("ENABLED","ENABLED_BY_DEFAULT") | ` Sort-Object RegionName).RegionName ForEach ($Region in $EnabledRegions) { Write-Host("`tRegion: $Region") # Get snashots. Write-Host ("`t`tRetrieving snapshots...") $Snapshots = Get-EC2Snapshot -ProfileName $Profile ` -Region $Region ` -OwnerId self If ($Snapshots) { # Get volumes. Write-Host ("`t`tRetrieving volumes...") $Volumes = Get-EC2Volume -ProfileName $Profile -Region $Region # Get EC2s Write-Host ("`t`tRetrieving EC2s...") $EC2s = (Get-EC2Instance -ProfileName $Profile -Region $Region).Instances Write-Host("`t`tsnapshots count: $($Snapshots.Count)") # For each snapshot: $Count = 1 ForEach ($Snapshot in $Snapshots) { Write-Host ("`t`t`t$Count- $($Snapshot.SnapshotId)") # Get Volume information. If ($Count -eq 4) { break } $Volume = $Volumes | Where {$_.VolumeId -eq $Snapshot.VolumeId} If ($Volume) { $VolumeExists = "Yes" $VolumeName = ($Volume.Tag | Where -Property Key -eq Name).Value $VolumeStatus = $Volume.Status.Value # Get attached EC2 information. If ($Volume.Attachment) { $EC2 = $EC2s | Where {$_.InstanceId -eq $Volume.Attachments.InstanceId} $EC2InstanceId = $EC2.InstanceId $EC2Name = ($EC2.Tag | Where -Property Key -eq Name).Value $EC2State = (Get-Culture).TextInfo.ToTitleCase($EC2.State.Name) } } Else { $EC2InstanceId = "" $EC2Name = "" $EC2State = "" $VolumeExists = "No" $VolumeName = "" $VolumeStatus = "" } $Info += $Snapshot | Select @{N="Profile";E={$Profile}}, @{N="Region";E={$Region}}, @{N="Name";E={($_.Tag | Where -Property Key -eq Name).Value}}, Encrypted, VolumeId, @{N="VolumeName";E={$VolumeName}}, @{N="VolumeStatus";E={$VolumeStatus}}, @{N="EC2InstanceId";E={$VolumeStatus}}, @{N="EC2Name";E={$EC2Name}}, @{N="EC2State";E={$EC2State}} $Count++ } # $Snapshot } Else { Write-Host("`t`tsnapshots count: 0") } } # $Region } $Info | Export-Csv -NoTypeInformation -Path $CsvFile <# $info - Requested EBS snapshot information across all profiles and enabled regions Profile: Production Region: us-east-1 Retrieving snapshots... Retrieving volumes... Retrieving EC2s... snapshots count: 4 1- snap-0d04d22c123456789 2- snap-0d04d22c123456781 3- snap-0d04d22c123456782 4- snap-0d04d22c123456783 Region: us-east-1 Retrieving snapshots... snapshots count: 0 #> |
Conclusion
Congratulations on reaching the end of this blog! Despite potentially challenging or lengthy code, you’ve made it through. Well done!
I utilized PowerShell and AWS Tools for PowerShell to create a script that retrieves all AWS EBS snapshots across all profiles and regions, including associated volume and EC2 information when available. You have the option to display the results, export them to a file, or both.
Did you find this blog easy to follow and helpful? I would love to hear your feedback and suggestions. Please let me know in the comments below.
Disclaimer
Purpose of the code contained in blog is solely for learning and demo purposes. Author will not be held responsible for any failure or damages caused due to any other usage.
There's no comments