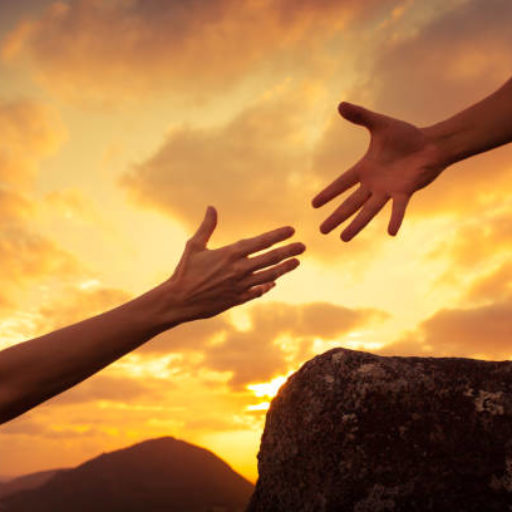
Azure App Registrations – Export certificates and secrets details
Introduction
This PowerShell function returns information about Azure App Registrations certificates and secrets, including expiry date, days left to expire, owner and other information.
Returned information can be filtered, for example, by days left to expire and imported to CSV.
Commands
Add-Member | Adds custom properties and methods to an instance of a PowerShell object. |
Get-MgApplication | Get the properties and relationships of an application object. |
Get-MgApplicationOwner | Directory objects that are owners of the application. |
New-Object | Creates an instance of a Microsoft .NET Framework or COM object. |
Function
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 |
function Get-MgApplicationCertificatesSecretsInfoS { <# .SYNOPSIS Returns a list of Azure App Registration with information about their certificas and secrets. .DESCRIPTION Get-MgApplicationCertificatesSecretsInfoS is a function that returns a list of all or selected Azure App Registration with information about their certificas and/or secrets. .PARAMETER Entity What to include in the returned list. Value: "Certificates", "Secrets" or "Certificates,Secrets" Default value: "Certificates,Secrets" .PARAMETER Filter Filter to include in Get-MgApplication. Example: "DisplayName eq 'Connect to Dynamics'" Example: "AppId eq '39b09640-ec3e-44c9-b3de-f52db4e1cf66'" .EXAMPLE Get-MgApplicationCertificatesSecretsInfoS | FT -AutoSize Returns all Azure Application Registrations that have certificates, secrets or both. .EXAMPLE Get-MgApplicationCertificatesSecretsInfoS | Where {$_.DaysLeft -lt 0} | FT -AutoSize Returns all Azure Application Registrations that have certificates, secrets or both with Daysleft less than zero, expired. .EXAMPLE Get-MgApplicationCertificatesSecretsInfoS | Export-CSV -NoTypeInformation -Path "C:\Temp\AzureAppRegCertSecretsInfo.csv" Returns all Azure Application Registrations that have certificates, secrets or both and save them to CSV file. .EXAMPLE Get-MgApplicationCertificatesSecretsInfoS -Verbose -Entity "Certificates" -Filter "DisplayName eq 'Connect to Dynamics'" | FT -AutoSize Returns certificates for Azure Application Registration named 'Connect to Dynamics'. .EXAMPLE "Secrets" | Get-MgApplicationCertificatesSecretsInfoS -Verbose -Filter "DisplayName eq 'Connect to Dynamics'" | FT -AutoSize Returns Secrets for Azure Application Registration named 'Connect to Dynamics'. .INPUTS String .OUTPUTS PSCustomObject ApplicationName, ApplicationID, Type, Description, StartDate, EndDate, DaysLeft, KeyId and Owners .NOTES Author: Saad Khamis Website: https://saadkhamis.com/azure-app-registrations-export-certificates-and-secrets-details/ #> [CmdletBinding()] param ( [Parameter(ValueFromPipeline)] [ValidateNotNullOrEmpty()] [string] $Entity = "Certificates,Secrets", [string] $Filter = $null ) BEGIN { $Param = if ($Filter -eq $null) { @{ "All" = $true } } else { @{ Filter = $Filter } } $Apps = Get-MgApplication @Param | Sort-Object DisplayName $now = get-date $Returns = @() } PROCESS { $Entity = $Entity.ToLower() $Entity = $Entity.Replace("certificates", "KeyCredentials") $Entity = $Entity.Replace("secrets", "PasswordCredentials") Write-Verbose -Message "" ForEach ($App in $Apps) { Write-Verbose -Message "$($App.DisplayName)" $owner = Get-MgApplicationOwner -ApplicationId $App.Id foreach ($EntityName in $Entity.Split(",")) { Write-Verbose -Message "`tProcessing $Type" $Type = if ($EntityName -eq "KeyCredentials") { "Certificate" } else { "Secret" } foreach ($EntityObj in $App.($EntityName)) { Write-Verbose -Message "`t`tName [$($EntityObj.DisplayName)], Id [$($EntityObj.KeyId)]" $DaysLeft = ($EntityObj.EndDateTime - $now).Days $Return = New-Object System.Object $Return | Add-Member -MemberType NoteProperty -Name "ApplicationName" -Value $App.DisplayName $Return | Add-Member -MemberType NoteProperty -Name "ApplicationID" -Value $App.Id $Return | Add-Member -MemberType NoteProperty -Name "Type" -Value $Type $Return | Add-Member -MemberType NoteProperty -Name "Description" -Value $EntityObj.DisplayName $Return | Add-Member -MemberType NoteProperty -Name "StartDate" -Value $EntityObj.StartDateTime $Return | Add-Member -MemberType NoteProperty -Name "EndDate" -value $EntityObj.EndDateTime $Return | Add-Member -MemberType NoteProperty -Name "DaysLeft" -value $DaysLeft $Return | Add-Member -MemberType NoteProperty -Name "KeyId" -value $EntityObj.KeyId $Return | Add-Member -MemberType NoteProperty -Name "Owners" -Value $owner.AdditionalProperties.userPrincipalName $Returns += $Return } } } } END { return ($Returns) } } # Get-MgApplicationCertificatesSecretsInfoS |
Examples
1 |
Connect-MgGraph -Scopes 'Application.Read.All' |
You must first call Connect-MgGraph command to sign in with the required scopes.
1 |
Get-MgApplicationCertificatesSecretsInfoS | FT -AutoSize |
1. Returns all Azure Application Registrations that have certificates, secrets or both.
1 |
Get-MgApplicationCertificatesSecretsInfoS | Where {$_.DaysLeft -lt 0} | FT -AutoSize |
2. Returns all Azure Application Registrations that have certificates, secrets or both with Daysleft less than zero, expired.
1 |
Get-MgApplicationCertificatesSecretsInfoS | Export-CSV -NoTypeInformation -Path "C:\Temp\AzureAppRegCertSecretsInfo.csv" |
3. Returns all Azure Application Registrations that have certificates, secrets or both and save them to CSV file.
1 |
Get-MgApplicationCertificatesSecretsInfoS -Verbose -Entity "Certificates" -Filter "DisplayName eq 'Connect to Dynamics'" | FT -AutoSize |
4. Returns certificates for Azure Application Registration named ‘Connect to Dynamics’.
1 |
"Secrets" | Get-MgApplicationCertificatesSecretsInfoS -Verbose -Filter "DisplayName eq 'Connect to Dynamics'" | FT -AutoSize |
5. Returns Secrets for Azure Application Registration named ‘Connect to Dynamics’.
Function Explanation
Functions accepts two parameters:
- Entity: Set it to “Certificates”, “Secrets” or “Certificates,Secrets”.
- Filter: (Optional) to include in Get-MgApplication.
Entity is set as parameter which can take values from the incoming pipeline object, example 5.
Entity values are converted to value that is used to acquire the required values:
- Certificates -> KeyCredentials.
- Secrets -> PasswordCredentials.
New-Object and Add-Object are used to create a custom object.
In conclusion
In summary, we explored how to get information about Azure App Registrations certificates and secrets.
Did you find this function easy to follow and helpful to you? I certainly would love to hear your feedback and suggestions. So, let me know in the comments below. Happy PowerShelling.
Disclaimer
Purpose of the code contained in blog is solely for learning and demo purposes. Author will not be held responsible for any failure or damages caused due to any other usage.
There's no comments